Linux firewalling Threat intelligence script
This is a threat intelligence script to use on a linux based network aggregator. It is based on this one.
This is a threat intelligence script to use on a linux based network aggregator. It is based on this one.
First install the prerequisites.
sudo apt-get install ipset iprange
Place the file updatethreatblock in the directory /usr/local/sbin.
#!/usr/bin/env bash## usage updatethreatblock.sh <configuration file># eg: updatethreatblock.sh /etc/ipset-threatblock/ipset-threatblock.conf#function exists() { command -v "$1" >/dev/null 2>&1 ; }if [[ -z "$1" ]]; thenecho "Error: please specify a configuration file, e.g. $0 /etc/ipset-threatblock/ipset-threatblock.conf"exit 1fi# shellcheck source=ipset-threatblock.confif ! source "$1"; thenecho "Error: can't load configuration file $1"exit 1fiif ! exists curl && exists egrep && exists grep && exists ipset && exists iptables && exists sed && exists sort && exists wc ; thenecho >&2 "Error: searching PATH fails to find executables among: curl egrep grep ipset iptables sed sort wc"exit 1fiDO_OPTIMIZE_CIDR=noif exists iprange && [[ ${OPTIMIZE_CIDR:-yes} != no ]]; thenDO_OPTIMIZE_CIDR=yesfiif [[ ! -d $(dirname "$IP_BLACKLIST") || ! -d $(dirname "$IP_BLACKLIST_RESTORE") ]]; thenecho >&2 "Error: missing directory(s): $(dirname "$IP_BLACKLIST" "$IP_BLACKLIST_RESTORE"|sort -u)"exit 1fiif [ -f "$IP_BLACKLIST_EXCEPTIONS" ]; thenEXCEPTIONS_TMP=$(mktemp)for exception in $(sed -r -e 's/\s*#.*$//;/^$/d;/^(0.0.0.0|10.|127.|172.1[6-9].|172.2[0-9].|172.3[0-1].|192.168.|22[4-9].|23[0-9].)/d' "$IP_BLACKLIST_EXCEPTIONS")doexception_array+=( "$exception" )echo $exception >> $EXCEPTIONS_TMPdonefi# create the ipset if needed (or abort if does not exists and FORCE=no)if ! ipset list -n|command grep -q "$IPSET_BLACKLIST_NAME"; thenif [[ ${FORCE:-no} != yes ]]; thenecho >&2 "Error: ipset does not exist yet, add it using:"echo >&2 "# ipset create $IPSET_BLACKLIST_NAME -exist hash:net family inet hashsize ${HASHSIZE:-16384} maxelem ${MAXELEM:-65536}"exit 1fiif ! ipset create "$IPSET_BLACKLIST_NAME" -exist hash:net family inet hashsize "${HASHSIZE:-16384}" maxelem "${MAXELEM:-65536}"; thenecho >&2 "Error: while creating the initial ipset"exit 1fifi# create the iptables binding if needed (or abort if does not exists and FORCE=no)if ! iptables -nvL INPUT|command grep -q "match-set $IPSET_BLACKLIST_NAME"; then# we may also have assumed that INPUT rule n°1 is about packets statistics (traffic monitoring)if [[ ${FORCE:-no} != yes ]]; thenecho >&2 "Error: iptables does not have the needed ipset INPUT rule, add it using:"echo >&2 "# iptables -I INPUT ${IPTABLES_IPSET_RULE_NUMBER:-1} -m set --match-set $IPSET_BLACKLIST_NAME src -j DROP"exit 1fiif ! iptables -I INPUT "${IPTABLES_IPSET_RULE_NUMBER:-1}" -m set --match-set "$IPSET_BLACKLIST_NAME" src -j DROP; thenecho >&2 "Error: while adding the --match-set ipset rule to iptables"exit 1fifiIP_BLACKLIST_TMP=$(mktemp)for i in "${BLACKLISTS[@]}"doIP_TMP=$(mktemp)(( HTTP_RC=$(curl -L -A "blacklist-update/script/github" --connect-timeout 10 --max-time 10 -o "$IP_TMP" -s -w "%{http_code}" "$i") ))if (( HTTP_RC == 200 || HTTP_RC == 302 || HTTP_RC == 0 )); then # "0" because file:/// returns 000command grep -Po '^(?:\d{1,3}\.){3}\d{1,3}(?:/\d{1,2})?' "$IP_TMP" | sed -r 's/^0*([0-9]+)\.0*([0-9]+)\.0*([0-9]+)\.0*([0-9]+)$/\1.\2.\3.\4/' >> "$IP_BLACKLIST_TMP"[[ ${VERBOSE:-yes} == yes ]] && echo -n "."elif (( HTTP_RC == 503 )); thenecho -e "\\nUnavailable (${HTTP_RC}): $i"elseecho >&2 -e "\\nWarning: curl returned HTTP response code $HTTP_RC for URL $i"firm -f "$IP_TMP"done# sort -nu does not work as expectedsed -r -e '/^(0\.0\.0\.0|10\.|127\.|172\.1[6-9]\.|172\.2[0-9]\.|172\.3[0-1]\.|192\.168\.|22[4-9]\.|23[0-9]\.)/d' "$IP_BLACKLIST_TMP"|sort -n|sort -mu >| "$IP_BLACKLIST"if [[ ${DO_OPTIMIZE_CIDR} == yes ]]; thenif [[ ${VERBOSE:-no} == yes ]]; thenecho -e "\\nAddresses before CIDR optimization: $(wc -l "$IP_BLACKLIST" | cut -d' ' -f1)"fi< "$IP_BLACKLIST" iprange --optimize - > "$IP_BLACKLIST_TMP" 2>/dev/nullif [[ ${#exception_array[@]} > 0 ]]; thenecho "Allowing for ${#exception_array[@]} exclusions from blacklist"echo "Addresses before removing exclusions: $(wc -l "$IP_BLACKLIST_TMP" | cut -d' ' -f1)"IP_BLACKLIST_WITH_EXCEPT_TMP=$(mktemp)iprange "$IP_BLACKLIST_TMP" --except "$EXCEPTIONS_TMP" > "$IP_BLACKLIST_WITH_EXCEPT_TMP" 2>/dev/nullcp "$IP_BLACKLIST_WITH_EXCEPT_TMP" "$IP_BLACKLIST_TMP"fiif [[ ${VERBOSE:-no} == yes ]]; thenecho "Addresses after CIDR optimization: $(wc -l "$IP_BLACKLIST_TMP" | cut -d' ' -f1)"ficp "$IP_BLACKLIST_TMP" "$IP_BLACKLIST"firm -f "$IP_BLACKLIST_TMP"# family = inet for IPv4 onlycat >| "$IP_BLACKLIST_RESTORE" <<EOFcreate $IPSET_TMP_BLACKLIST_NAME -exist hash:net family inet hashsize ${HASHSIZE:-16384} maxelem ${MAXELEM:-65536}create $IPSET_BLACKLIST_NAME -exist hash:net family inet hashsize ${HASHSIZE:-16384} maxelem ${MAXELEM:-65536}EOF# can be IPv4 including netmask notation# IPv6 ? -e "s/^([0-9a-f:./]+).*/add $IPSET_TMP_BLACKLIST_NAME \1/p" \ IPv6sed -rn -e '/^#|^$/d' \-e "s/^([0-9./]+).*/add $IPSET_TMP_BLACKLIST_NAME \\1/p" "$IP_BLACKLIST" >> "$IP_BLACKLIST_RESTORE"cat >> "$IP_BLACKLIST_RESTORE" <<EOFswap $IPSET_BLACKLIST_NAME $IPSET_TMP_BLACKLIST_NAMEdestroy $IPSET_TMP_BLACKLIST_NAMEEOFipset -file "$IP_BLACKLIST_RESTORE" restoreif [[ ${VERBOSE:-no} == yes ]]; thenechoecho "Threatblock addresses found: $(wc -l "$IP_BLACKLIST" | cut -d' ' -f1)"fi
Make the script executable: sudo chmod +x /usr/local/sbin/updatethreatblock.sh
Create a directory for the config files: sudo mkdir /etc/ipset-threatblock
Create a direct for the log files: /var/log/threatblock
Create a configuration file ipset-threatblock.conf in /etc/ipset-threatblock
IPSET_BLACKLIST_NAME=threatblock # change it if it collides with a pre-existing ipset listIPSET_TMP_BLACKLIST_NAME=${IPSET_BLACKLIST_NAME}-tmp# ensure the directory for IP_BLACKLIST/IP_BLACKLIST_RESTORE exists (it won't be created automatically)IP_BLACKLIST_RESTORE=/etc/ipset-threatblock/ip-threatblock.restoreIP_BLACKLIST=/etc/ipset-threatblock/ip-threatblock.listIP_BLACKLIST_EXCEPTIONS=/etc/ipset-threatblock/threatblock.exceptionsVERBOSE=yes # probably set to "no" for cron jobs, default to yesFORCE=yes # will create the ipset-iptable binding if it does not already existlet IPTABLES_IPSET_RULE_NUMBER=1 # if FORCE is yes, the number at which place insert the ipset-match rule (default to 1)# Sample (!) list of URLs for IP blacklists. Currently, only IPv4 is supported in this script, everything else will be filtered.BLACKLISTS=("file:///etc/ipset-blacklist/ip-threatblock-custom.list" # optional, for your personal nemeses (no typo, plural)"https://www.projecthoneypot.org/list_of_ips.php?t=d&rss=1" # Project Honey Pot Directory of Dictionary Attacker IPs"https://check.torproject.org/cgi-bin/TorBulkExitList.py?ip=1.1.1.1" # TOR Exit Nodes"http://danger.rulez.sk/projects/bruteforceblocker/blist.php" # BruteForceBlocker IP List"https://www.spamhaus.org/drop/drop.lasso" # Spamhaus Don't Route Or Peer List (DROP)"https://cinsscore.com/list/ci-badguys.txt" # C.I. Army Malicious IP List"https://lists.blocklist.de/lists/all.txt" # blocklist.de attackers"https://blocklist.greensnow.co/greensnow.txt" # GreenSnow"https://raw.githubusercontent.com/firehol/blocklist-ipsets/master/firehol_level1.netset" # Firehol Level 1"https://raw.githubusercontent.com/firehol/blocklist-ipsets/master/stopforumspam_7d.ipset" # Stopforumspam via Firehol"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_shadowserver.txt" # Shadow server"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_shodan.txt" # Shodan"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_sogou.txt" # Sogou search engine"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_evil.txt" # Hostile IPs"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_internet_cens.txt" # Internet census"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_diverseenvironment.txt" # Diverse environment"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_netsysres.txt" # Net Systems Research"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_rwth-aachen.txt" # AAChen"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_onyphe.txt" # onephe"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_stretchoid.txt" # Strechoid"https://gitlab.com/ohisee/block-shodan-stretchoid-census/raw/master/pf_table_openportsstats.txt" # OpenPortStats# "http://ipverse.net/ipblocks/data/countries/xx.zone" # Ban an entire country, see http://ipverse.net/ipblocks/data/countries/)MAXELEM=131072
Use a threatblock.exceptions file in /etc/ipset-threatblock to overwrite blocks IPs.
Use a ip-threatblock-custom.list file in /etc/ipset-threatblock to add custom IPs for blocking.
Create a script startubl.sh in /usr/local/sbin to use in crontab
#!/bin/bashPATH=/usr/local/sbin:/usr/local/bin:/sbin:/bin:/usr/sbin:/usr/bincd /usr/local/sbindate > /var/log/threatblock/tb-`date +%d%H`.run/usr/local/sbin/updatethreatblock.sh /etc/ipset-threatblock/ipset-threatblock.confdate >> /var/log/threatblock/tb-`date +%d%H`.run
Create a script rebootubl.sh in /usr/local/sbin
#!/bin/bash# Threat intelligence/sbin/ipset restore < /etc/ipset-threatblock/ip-threatblock.restore/sbin/iptables -I INPUT 1 -i enp4s0f1 -m set --match-set threatblock src -j DROP -m comment --comment "threat intel"/sbin/iptables -I FORWARD 1 -i enp4s0f1 -m set --match-set threatblock src -j DROP -m comment --comment "threat intel"/sbin/iptables -I FORWARD 1 -o enp4s0f1 -m set --match-set threatblock src -j DROP -m comment --comment "threat intel"
Update crontab: sudo crontab -e
## m h dom mon dow command@reboot sleep 180 && /usr/local/sbin/rebootubl.sh@daily /usr/local/sbin/startubl.sh
FROM: https://www.linkedin.com/pulse/threat-intelligence-script-ronald-bartels?trk=articles_directory
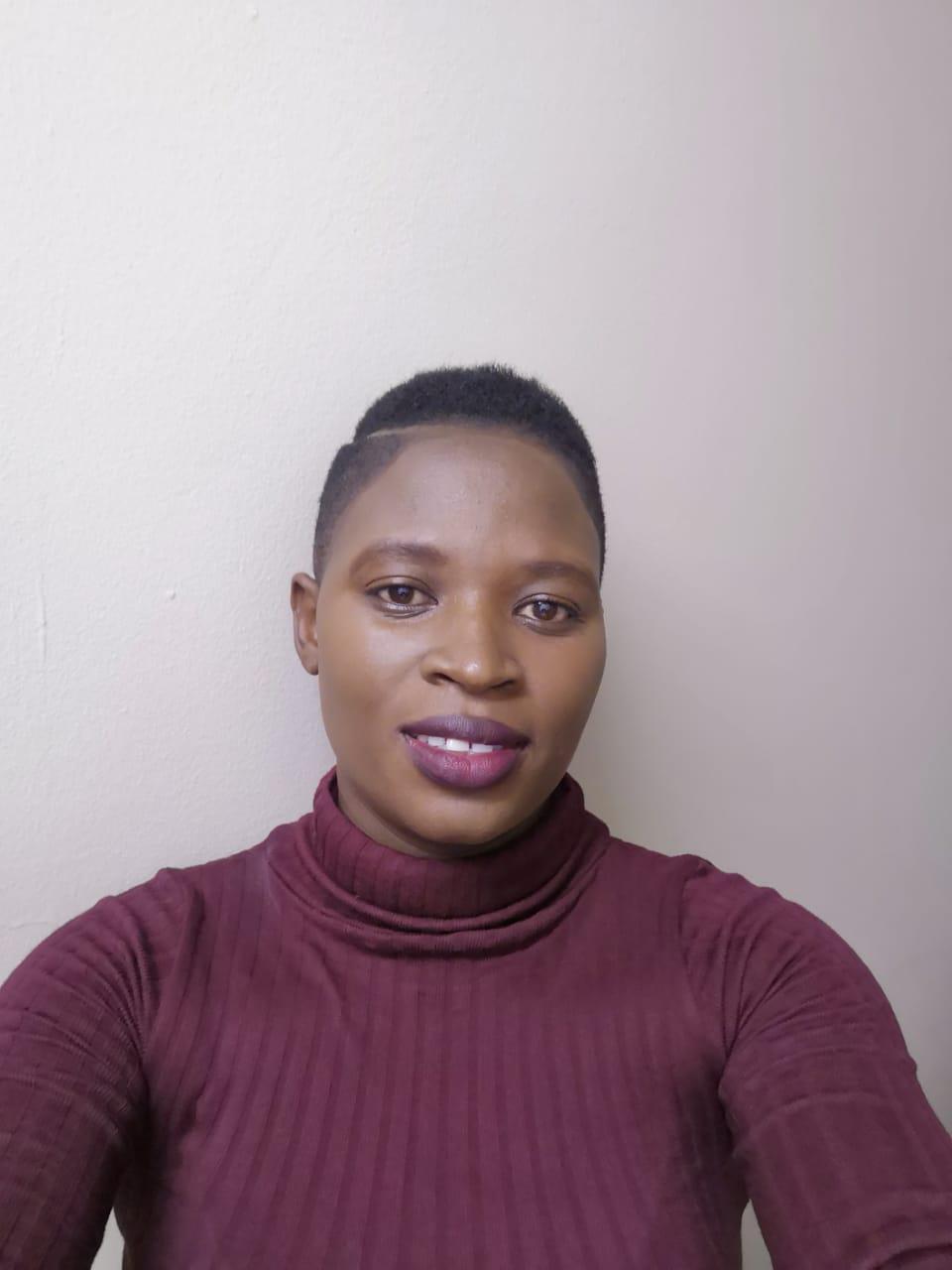
Carol Kamuchira
Digital Marketing Rep
No comments yet. Start a new discussion.